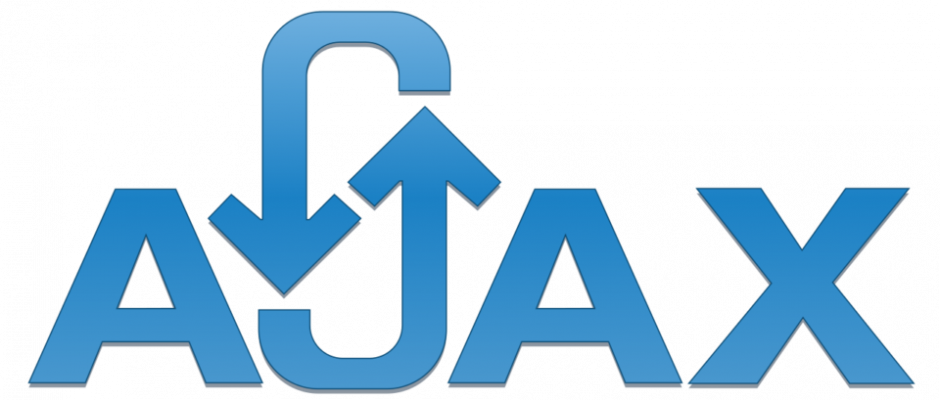
Display A Progress Bar Outputting The Progress Of a Long Running PHP Script
Sometimes you may have a PHP script that takes a while to run. To keep the user informed on the progress of the script, and to reassure the user that something is actually happening, it would be advisable do display a progress bar.
This can be achieved in a number of ways. One way is via ajax and the other way is via SSE (server side events). In this article we will be covering the ajax version. This is my preferred method as it is the methoad that is most supported by browsers. IE does not support currently SSE. If you are interested in learning more about SSE please read PHP Long script progress bar using SSE
JavaScript:
$( document ).ready(function() { var jsonResponse = '', lastResponseLen = false; $("form").submit(function( e ) { $('.ajax-res').slideDown(); $.ajax({ url: $(this).attr('action'), xhrFields: { onprogress: function(e) { var thisResponse, response = e.currentTarget.response; if(lastResponseLen === false) { thisResponse = response; lastResponseLen = response.length; } else { thisResponse = response.substring(lastResponseLen); lastResponseLen = response.length; } jsonResponse = JSON.parse(thisResponse); $('.ajax-res p').text('Processed '+jsonResponse.count+' of '+jsonResponse.total); $(".progress-bar").css('width', jsonResponse.progress+'%').text(jsonResponse.progress+'%'); } }, success: function(text) { console.log('done!'); $('.ajax-res p').text('Process completed successfully'); $(".progress-bar").css({ width:'100%', backgroundColor: 'green' }); } }); e.preventDefault(); }); });
Long Running PHP Script:
$total = 25; $i = 0; echo json_encode(array('progress' => 0, 'count' => $i, 'total' => $total)); flush(); ob_flush(); while ($i < $total) { $i++; echo json_encode(array('progress' => (($i/$total)*100), 'count' => $i, 'total' => $total)); flush(); ob_flush(); sleep(1); }
Thanks for reading!
Comments (0)